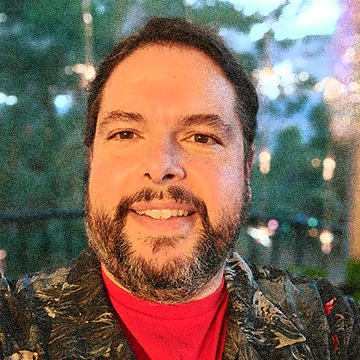
Ed Menendez
© 2024 All rights reserved.
Python Timezone Conversion Example Using pytz
Here's an example showing how to convert from one timezone to another in Python. Note that you won't be able to save the full date/time in MySQL without stripping out the timezone info.
from string import atoi
from datetime import datetime
import pytz # Available http://sourceforge.net/project/showfiles.php?group_id=79122
thedate = "20080518"
thetime = "2210"
europe_tz = pytz.timezone('Europe/Paris') # Note that your local install timezone should be settings.py
brazil_tz = pytz.timezone('America/Sao_Paulo')
server_tz = pytz.timezone('America/Los_Angeles')
stat_time = datetime(atoi(thedate[0:4]), atoi(thedate[4:6]), atoi(thedate[6:8]), atoi(thetime[0:2]), atoi(thetime[2:4]), 0, tzinfo=europe_tz)
stat_time.astimezone(brazil_tz) # returns time for brazil
stat_time.astimezone(server_tz) # returns server time