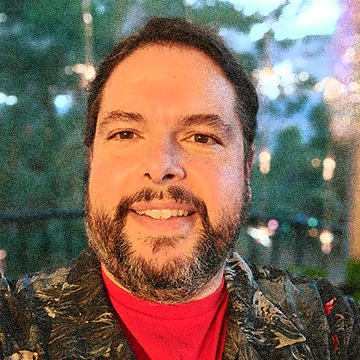
Ed Menendez
© 2024 All rights reserved.
Search Twitter Public Timeline with Python
This Python function takes a search string as a parameter and returns a dictionary representing the JSON returned by Twitter with your search results from the public timeline. Code requires simplejson. It supports all the parameters as defined by Twitter here.
def search_public_timeline(q, refresh_url=None, **kwargs):
'''
Searches the public timeline for the q string. There is no sanity checking
of the parameters. It's all passed straight to the API. Spec defined here:
http://apiwiki.twitter.com/Twitter-Search-API-Method:+search
If you send refresh_url then all parameters are ignored. Example usage:
search_public_timeline('menendez')
search_public_timeline('menendez', since='2010-04-15')
Ed Menendez - [email protected]
>>> len(search_public_timeline('the')['results']) > 1
True
'''
if not refresh_url:
parms = {}
parms['q'] = q
parms.update(kwargs)
query_str = '?%s' % urllib.urlencode(parms)
else:
query_str = refresh_url
u = 'http://search.twitter.com/search.json%s' % query_str
#user_agent = 'Mozilla/4.0 (compatible; MSIE 5.5; Windows NT)'
#headers = {'User-Agent' : user_agent}
req = urllib2.Request(u) #, None, headers
return simplejson.load(urllib2.urlopen(req))